OpenLayers で選択されたフィーチャのスタイルを変える方法をまとめました。
フィーチャの選択を検知し, 選択されたフィーチャのスタイルを変更します。
ライブラリ参照
- OpenLayers の js ライブラリとスタイルシートを参照します。
<link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/openlayers/openlayers.github.io@master/en/v6.4.3/css/ol.css" type="text/css">
<script src="https://cdn.jsdelivr.net/gh/openlayers/openlayers.github.io@master/en/v6.4.3/build/ol.js"></script>
画面
- 地図を描画する要素を用意します。
<div id="map"></div>
スクリプト
- ol.interaction.Select() でフィーチャを選択出来るようになります。
- interaction はマップに addInteraction() で追加する必要があります。
- getFeatures() で選択されたフィーチャを取得出来るので, スタイルを変える場合はフィーチャが選択された時のイベントハンドラにスタイルを変える処理を実装します。
function initializeSelect() {
var select = new ol.interaction.Select();
map.addInteraction(select);
var selectedFeatures = select.getFeatures();
selectedFeatures.on(['add', 'remove'], function () {
var selectedStyle = new ol.style.Style({
image: new ol.style.Circle({
radius: 8,
fill: new ol.style.Fill({ color: '#FF0000' }),
stroke: new ol.style.Stroke({ color: '#FFFFFF', width: 3 })
})
});
var features = selectedFeatures.getArray();
if (0 < features.length) {
features.map(f => { f.setStyle(selectedStyle); });
}
else {
console.log('no feature');
}
});
}
成果物
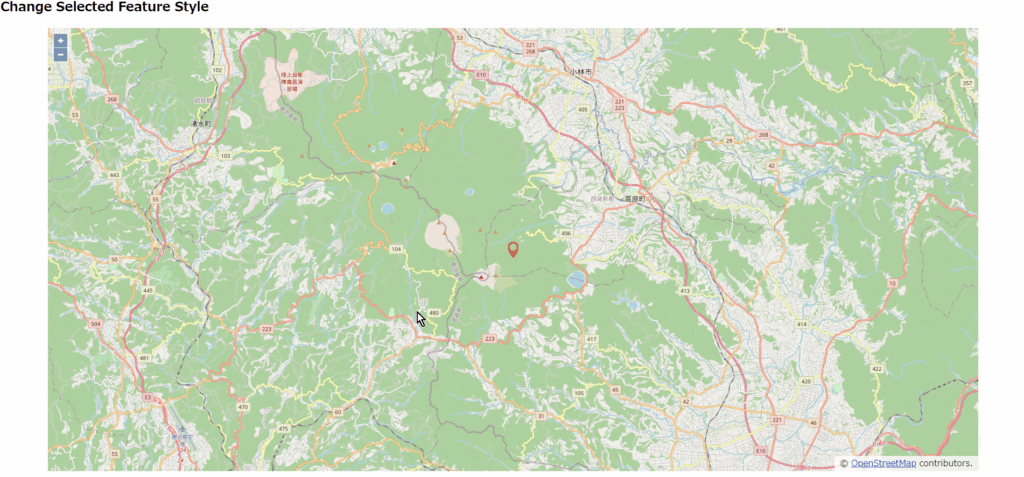
下記ソースコードを html ファイルとして保存し, Webブラウザで表示します。
<!doctype html>
<html lang="en">
<head>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/openlayers/openlayers.github.io@master/en/v6.4.3/css/ol.css"
type="text/css">
<style>
.map {
height: 85vh;
width: 90vw;
margin: auto;
}
</style>
<script src="https://cdn.jsdelivr.net/gh/openlayers/openlayers.github.io@master/en/v6.4.3/build/ol.js"></script>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script type="text/javascript">
var center = [130.93, 31.9];
var map;
$(function () {
initializeMap();
addIconFeature();
initializeSelect();
});
function initializeMap() {
const baseMap = new ol.layer.Tile({
source: new ol.source.OSM()
});
map = new ol.Map({
target: 'map',
layers: [baseMap],
view: new ol.View({
center: ol.proj.fromLonLat(center),
zoom: 12
})
});
}
function addIconFeature() {
var iconStyle = new ol.style.Style({
image: new ol.style.Icon({
src: 'icon.png'
})
});
var vectorLayer = new ol.layer.Vector({
source: new ol.source.Vector(),
style: iconStyle
});
var f = new ol.Feature(new ol.geom.Point(ol.proj.transform(center, 'EPSG:4326', 'EPSG:3857')));
f.setStyle(iconStyle);
vectorLayer.getSource().addFeature(f);
map.addLayer(vectorLayer);
}
function initializeSelect() {
var select = new ol.interaction.Select();
map.addInteraction(select);
var selectedFeatures = select.getFeatures();
selectedFeatures.on(['add', 'remove'], function () {
var selectedStyle = new ol.style.Style({
image: new ol.style.Circle({
radius: 8,
fill: new ol.style.Fill({ color: '#FF0000' }),
stroke: new ol.style.Stroke({ color: '#FFFFFF', width: 3 })
})
});
var features = selectedFeatures.getArray();
if (0 < features.length) {
features.map(f => { f.setStyle(selectedStyle); });
}
else {
console.log('no feature');
}
});
}
</script>
<title>Change Selected Feature Style</title>
</head>
<body>
<h2>Change Selected Feature Style</h2>
<div id="map" class="map"></div>
</body>
</html>